Summary -
In this topic, we described about the below sections -
The Id locating strategy searches for an element in the web page that having an id attribute corresponding to the specified value. According to the World Wide Web Consortium(W3C) ID should be unique to elements and considered as the fastest and safest method to locate element. So, Id attribute is a unique reference for an element in the web page that the developer sets while developing a web page. However, the browsers do allow exceptions to this rule. Developers may or may not follow this rule as browsers do allow bypassing this rule. i.e, a webpage may contain more than one element that are set to same ID attribute.
In selenium, the most popular way to identify web element is Id locator. Id’s are considered as the safest and fastest locator option when only ID value is unique on the webpage. If multiple elements exist with same Id attribute value, then first element matches the criteria uses for testing.
Pros
- Each id locator should be unique so no chance of matching several elements.
Cons
- Feasible only for elements with fixed ids and not generated ones.
Example
Now, let’s understand the working of ID locator with the help of a simple example. we will launch Chrome and navigate to bing.com. Here, we will try to locate the search box using ID Locator.
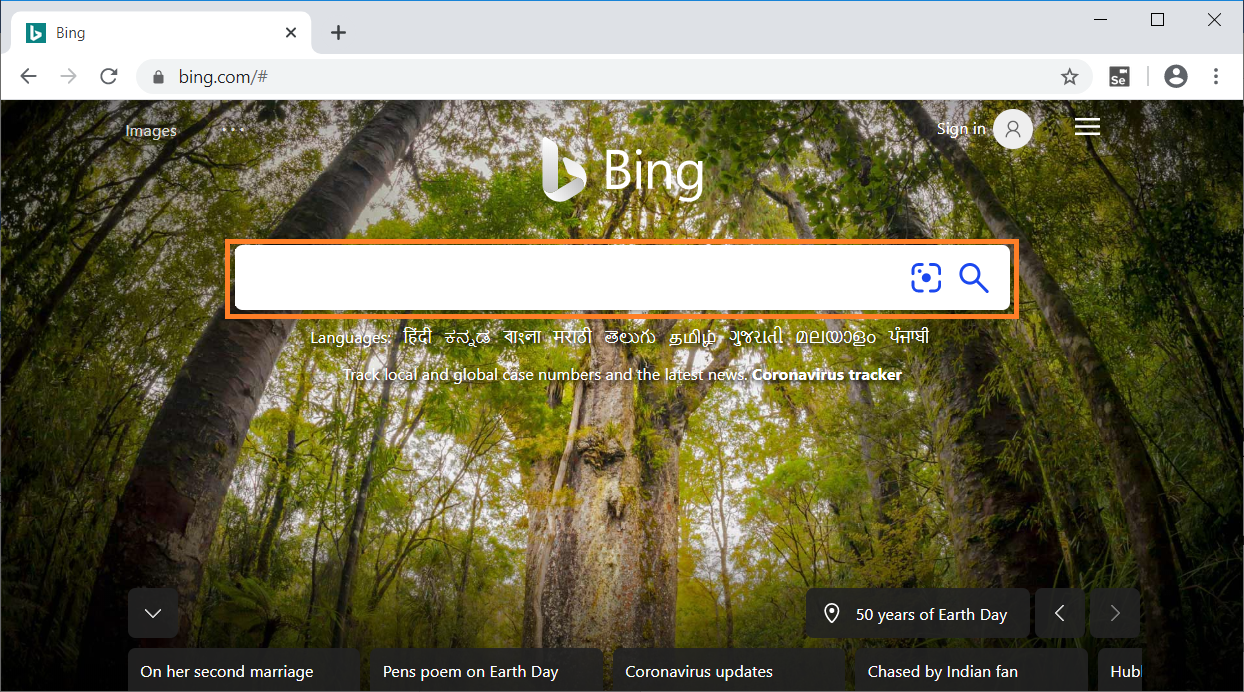
On inspecting the above web element, we can see an input tag has attribute id. Now, we will use the value of Id locator i.e. sb_form_q to perform search.

Let’s see how the automation of the search look like in Selenium IDE that send value “India” to the search box using Id locator.
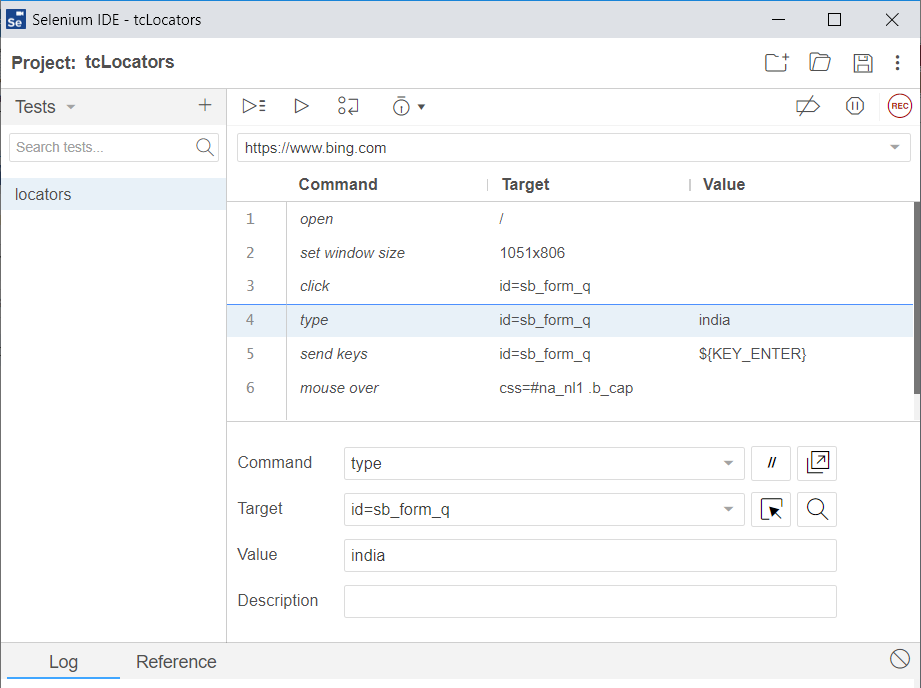
The java program for the above test is -
// Generated by Selenium IDE
import org.junit.Test;
import org.junit.Before;
import org.junit.After;
import static org.junit.Assert.*;
import static org.hamcrest.CoreMatchers.is;
import static org.hamcrest.core.IsNot.not;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.remote.RemoteWebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.Dimension;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.interactions.Actions;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.Alert;
import org.openqa.selenium.Keys;
import java.util.*;
import java.net.MalformedURLException;
import java.net.URL;
public class LocatorsTest {
private WebDriver driver;
private Map<String, Object> vars;
JavascriptExecutor js;
@Before
public void setUp() {
driver = new ChromeDriver();
js = (JavascriptExecutor) driver;
vars = new HashMap();
}
@After
public void tearDown() {
driver.quit();
}
@Test
public void locators() {
driver.get("https://www.bing.com/");
driver.manage().window().setSize(new Dimension(1051, 806));
driver.findElement(By.id("sb_form_q")).click();
driver.findElement(By.id("sb_form_q")).sendKeys("india");
driver.findElement(By.id("sb_form_q")).sendKeys(Keys.ENTER);
{
WebElement element=driver.findElement(
By.cssSelector("#na_nl1.b_cap"));
Actions builder = new Actions(driver);
builder.moveToElement(element).perform();
}
}
}
When you run the java program, Chrome driver launches chrome, redirect to bing search page,enter the search key word as “india” and navigate to search results page. Refer the below image for the output -
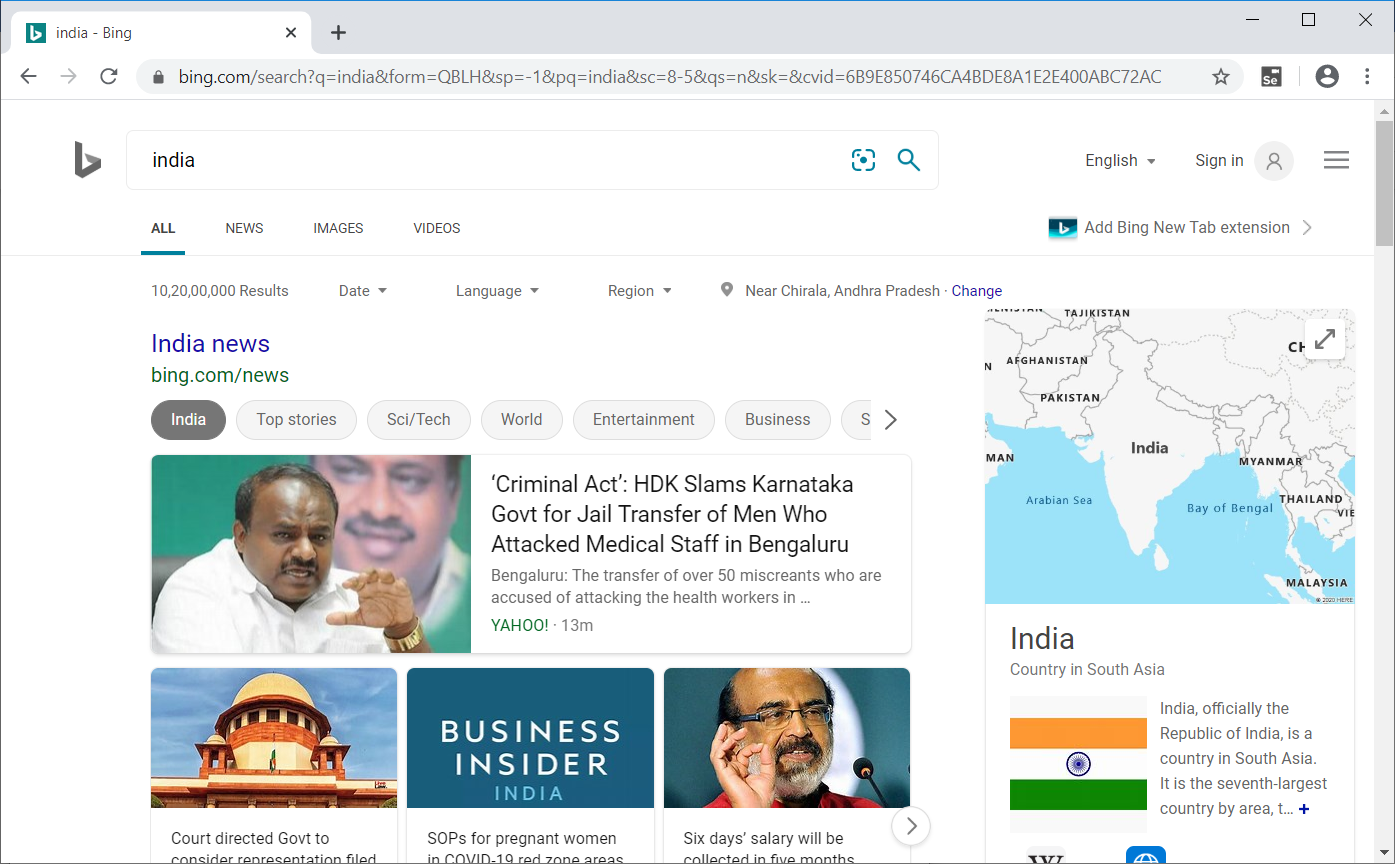
The above example gives a clear understanding of how Id locator in Selenium works.