Summary -
In this topic, we described about the below sections -
XPath used to locating elements on the web page XML documents or expressions. XPath is an important strategy to locate elements in selenium.
XPath also consists of a path expression along with some conditions. We can easily write an XPath script/query to locate any element in the webpage.
XPath is the standard navigation tool for XML and an HTML document is also an XML document (xHTML).
XPath is used everywhere where there is XML.
Attributes are defined via prefix ‘@’ and their corresponding value. There are multiple ways through which xpath can be defined and different attributes like name, id, class etc can be used.
XPath locators are robust and reliable. It is one method that guarantees to locate any element on the page using the XPath expression. However, we should be very careful while forming an XPath as it may not work if there are changes in the web application.
Pros
- Allows very accurate locators.
Cons
- Slow as compared to CSS.
- Relies on browser dependent that is not always complete.
- Is not recommended for cross-browser testing.
XPath Expressions Types
We can classify XPaths in to two groups -
- Absolute XPath
- Relative XPath
- Standard Xpath
- Using Contains
- Using Xpath with AND & OR
- Using starts-with o Using text in Xpath
Absolute XPath
Absolute path starts from the root element and goes to identify the target element within the web page.
To use locators like the XPath is easy when the direct element path provided. However, the XPath would break when the element structure changes.
For example, we can specify the path for table column like below –
html/head/body/table/tr/td
Example
Now, let’s understand the working of absolute xpath locator with the help of a simple example. I will launch Chrome and navigate to bing.com. Here, I will try to locate the search box using absolute xpath Locator.
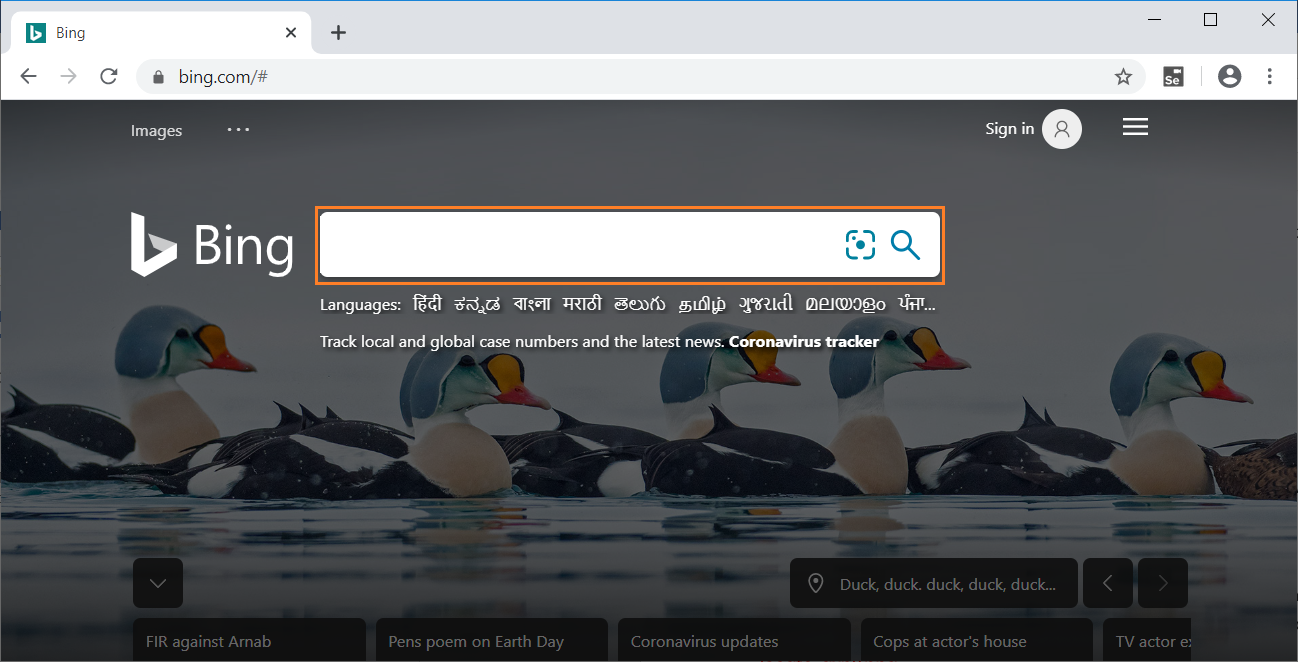
On inspecting the above web element, we can see an input tag has attribute id. Now, I will use the value of id i.e. "sb_form_q" along with absolute xpath locator to perform search.
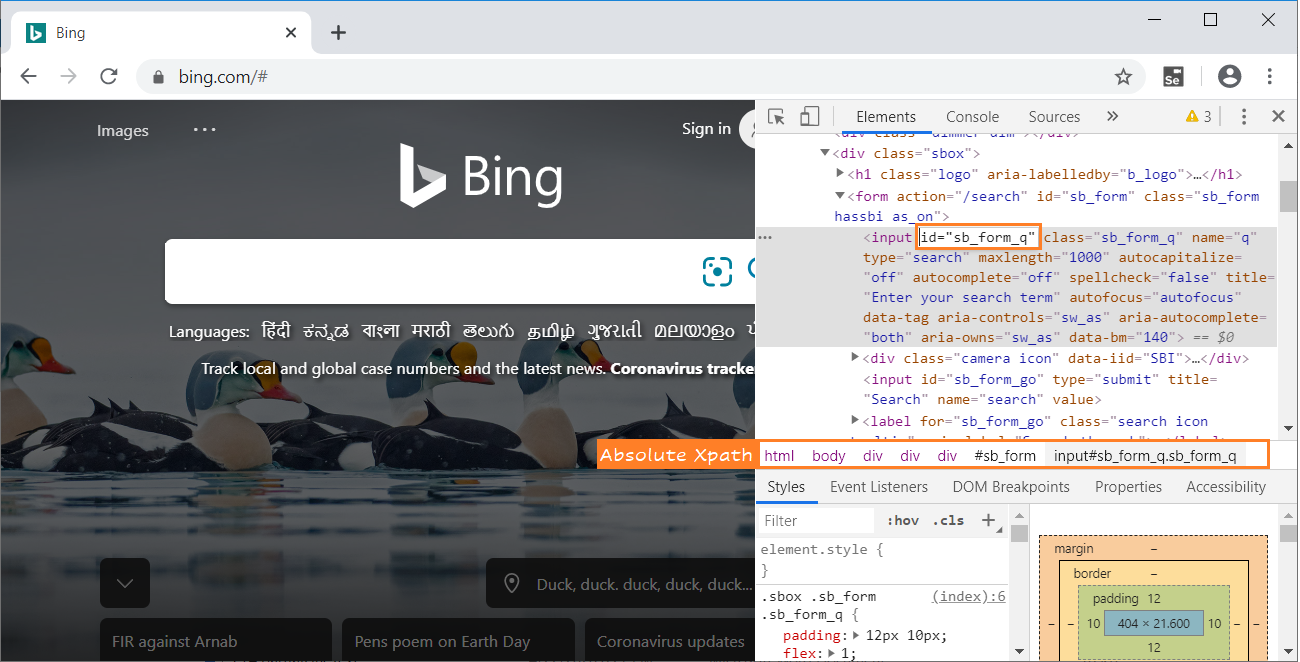
Let’s see how the automation of the search look like in Selenium IDE and send value "India" to the search box using absolute xpath locator.
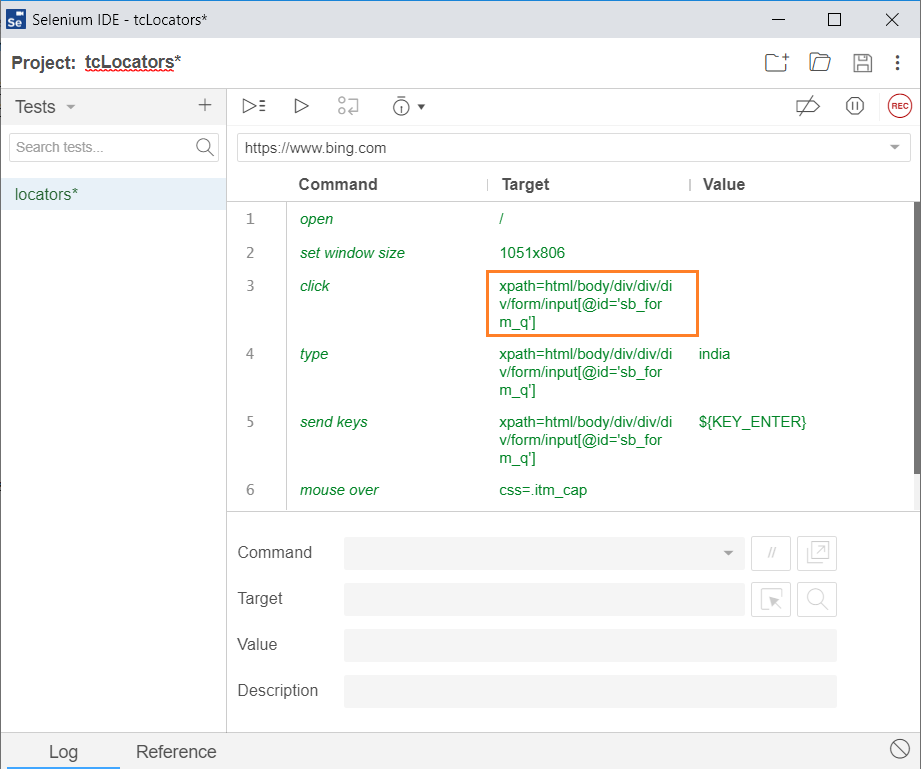
The java program for the above test is -
// Generated by Selenium IDE
import org.junit.Test;
import org.junit.Before;
import org.junit.After;
import static org.junit.Assert.*;
import static org.hamcrest.CoreMatchers.is;
import static org.hamcrest.core.IsNot.not;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.remote.RemoteWebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.Dimension;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.interactions.Actions;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.Alert;
import org.openqa.selenium.Keys;
import java.util.*;
import java.net.MalformedURLException;
import java.net.URL;
public class LocatorsTest {
private WebDriver driver;
private Map<String, Object> vars;
JavascriptExecutor js;
@Before
public void setUp() {
driver = new ChromeDriver();
js = (JavascriptExecutor) driver;
vars = new HashMap<String, Object>();
}
@After
public void tearDown() {
driver.quit();
}
@Test
public void locators() {
driver.get("https://www.bing.com/");
driver.manage().window().setSize(new Dimension(1051, 806));
driver.findElement(By.xpath(
"html/body/div/div/div/form/input[@id=\'sb_form_q\']")).
click();
driver.findElement(By.xpath(
"html/body/div/div/div/form/input[@id=\'sb_form_q\']")).
sendKeys("india");
driver.findElement(By.xpath(
"html/body/div/div/div/form/input[@id=\'sb_form_q\']")).
sendKeys(Keys.ENTER);
{
WebElement element = driver.findElement(
By.cssSelector(".itm_cap"));
Actions builder = new Actions(driver);
builder.moveToElement(element).perform();
}
}
}
When we run the java program, Chrome driver launches chrome, redirect to bing search page, enter the search key word as "india" and navigate to search results page. Refer the below image for the output -
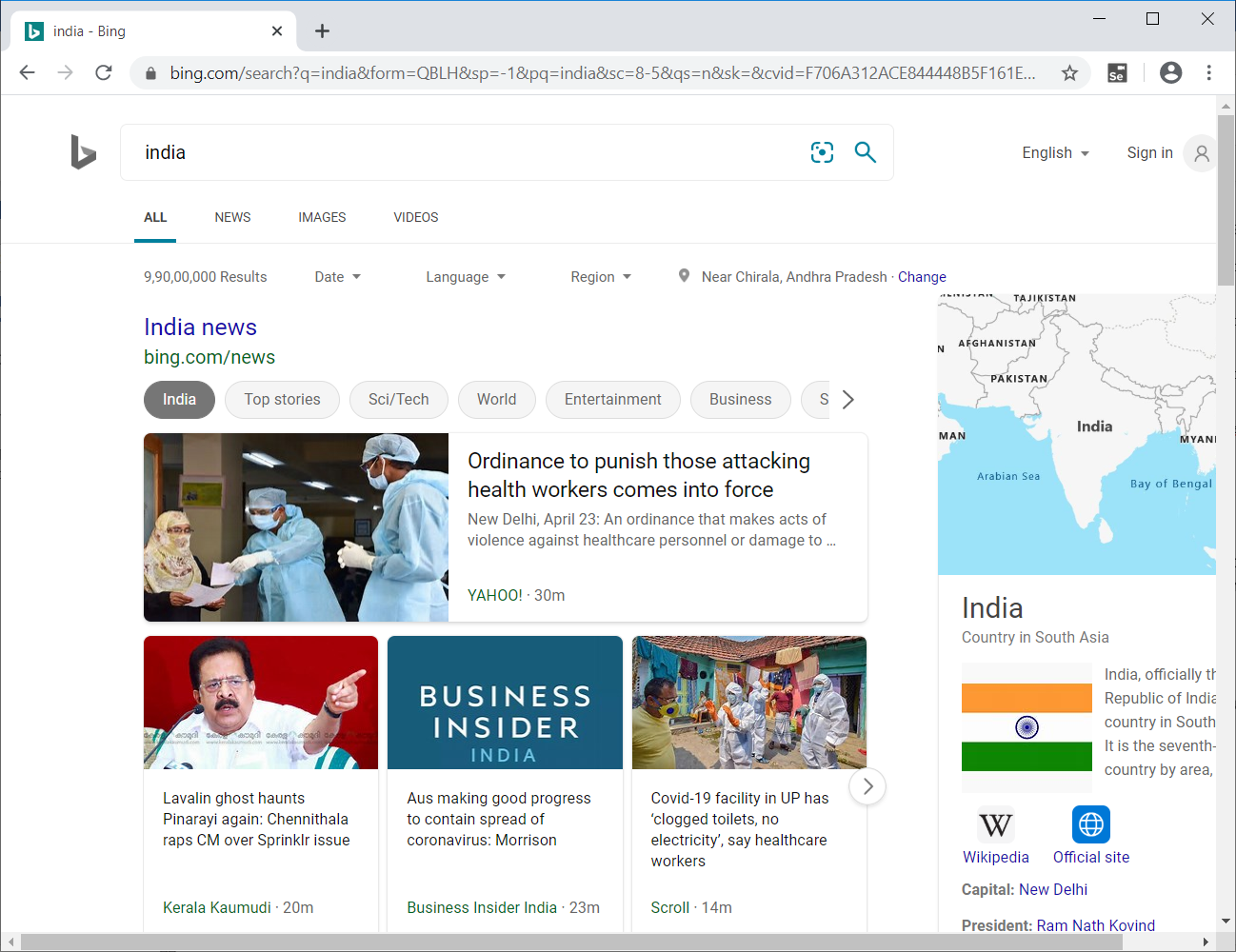
The above example gives a clear understanding of how absolute xpath locator in Selenium works.
Relative XPath
The relative XPath is very easy to manage as they are short and concise. It is better than the previous XPath style in terms of supplying html elements to the XPath. However, building a relative XPath is time-consuming and quite tricky. Because we need to check all the nodes to form the path.
For example, we can specify the path for table column like below –
//table/tr/td
Example
Now, let’s understand the working of relative xpath locator with the help of a simple example. I will launch Chrome and navigate to bing.com. Here, I will try to locate the search box using relative xpath Locator.
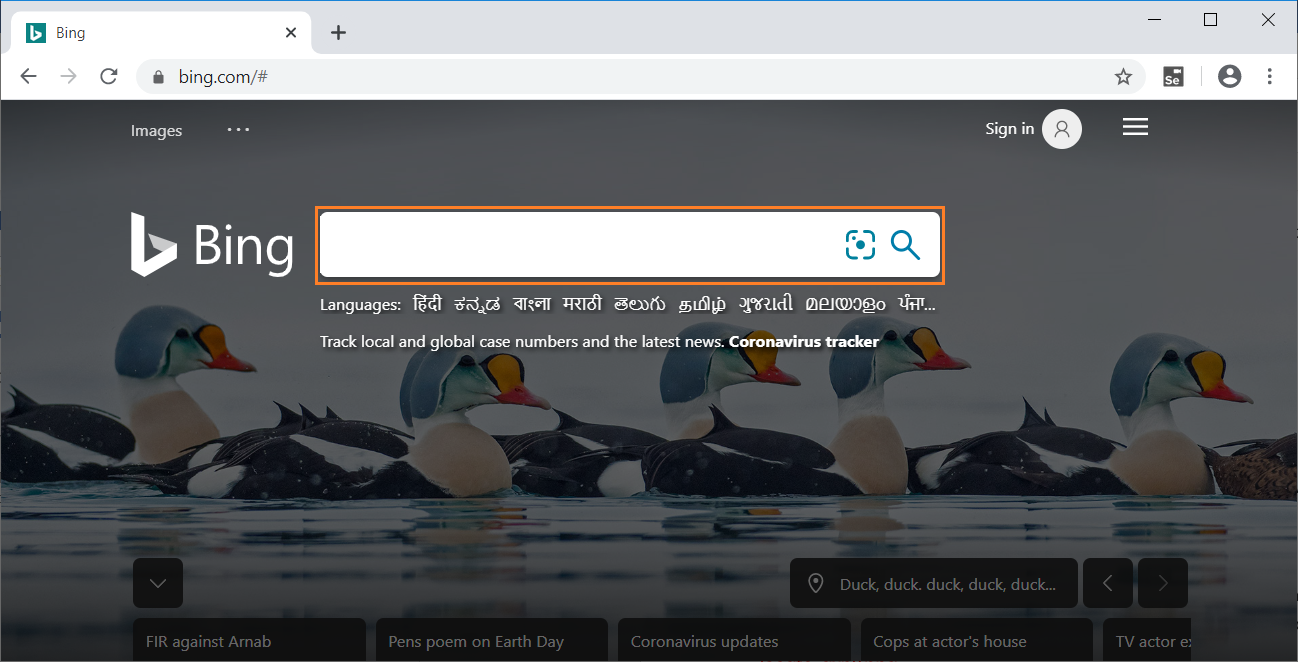
On inspecting the above web element, we can see an input tag has attribute id. Now, I will use the value of id i.e. "sb_form_q" along with relative xpath locator to perform search.
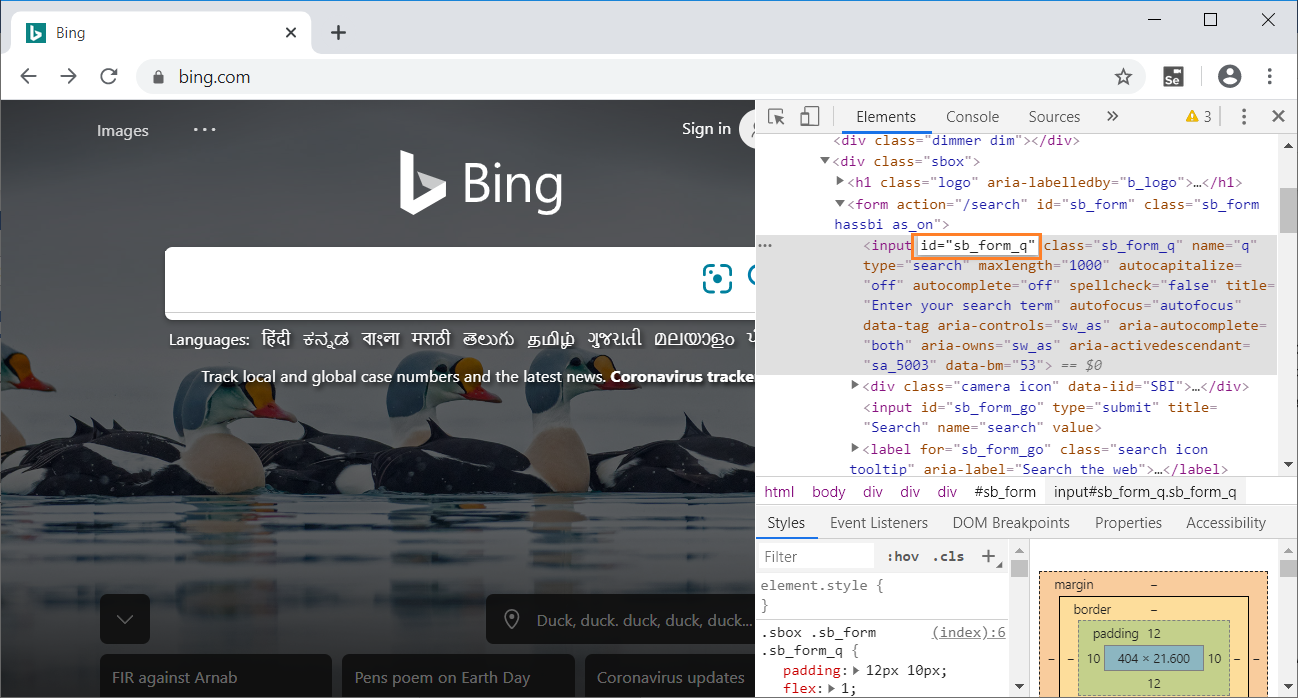
Let’s see how the automation of the search look like in Selenium IDE and send value "India" to the search box using id relative xpath locator.
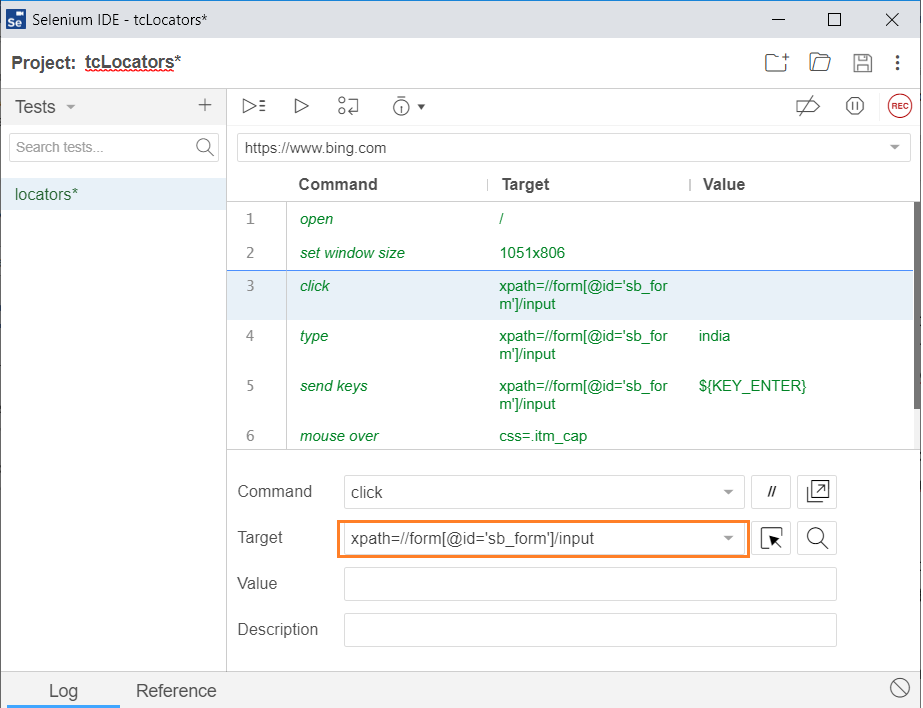
The java program for the above test is -
// Generated by Selenium IDE
import org.junit.Test;
import org.junit.Before;
import org.junit.After;
import static org.junit.Assert.*;
import static org.hamcrest.CoreMatchers.is;
import static org.hamcrest.core.IsNot.not;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.remote.RemoteWebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.Dimension;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.interactions.Actions;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.Alert;
import org.openqa.selenium.Keys;
import java.util.*;
import java.net.MalformedURLException;
import java.net.URL;
public class LocatorsTest {
private WebDriver driver;
private Map<String, Object> vars;
JavascriptExecutor js;
@Before
public void setUp() {
driver = new ChromeDriver();
js = (JavascriptExecutor) driver;
vars = new HashMap();
}
@After
public void tearDown() {
driver.quit();
}
@Test
public void locators() {
driver.get("https://www.bing.com/");
driver.manage().window().setSize(new Dimension(1051, 806));
driver.findElement(By.xpath(
"//form[@id=\'sb_form\']/input")).click();
driver.findElement(By.xpath(
"//form[@id=\'sb_form\']/input")).sendKeys("india");
driver.findElement(By.xpath(
"//form[@id=\'sb_form\']/input")).
sendKeys(Keys.ENTER);
{
WebElement element = driver.findElement(
By.cssSelector(".itm_cap"));
Actions builder = new Actions(driver);
builder.moveToElement(element).perform();
}
}
}
When we run the java program, Chrome driver launches chrome, redirect to bing search page, enter the search key word as "india" and navigate to search results page. Refer the below image for the output -
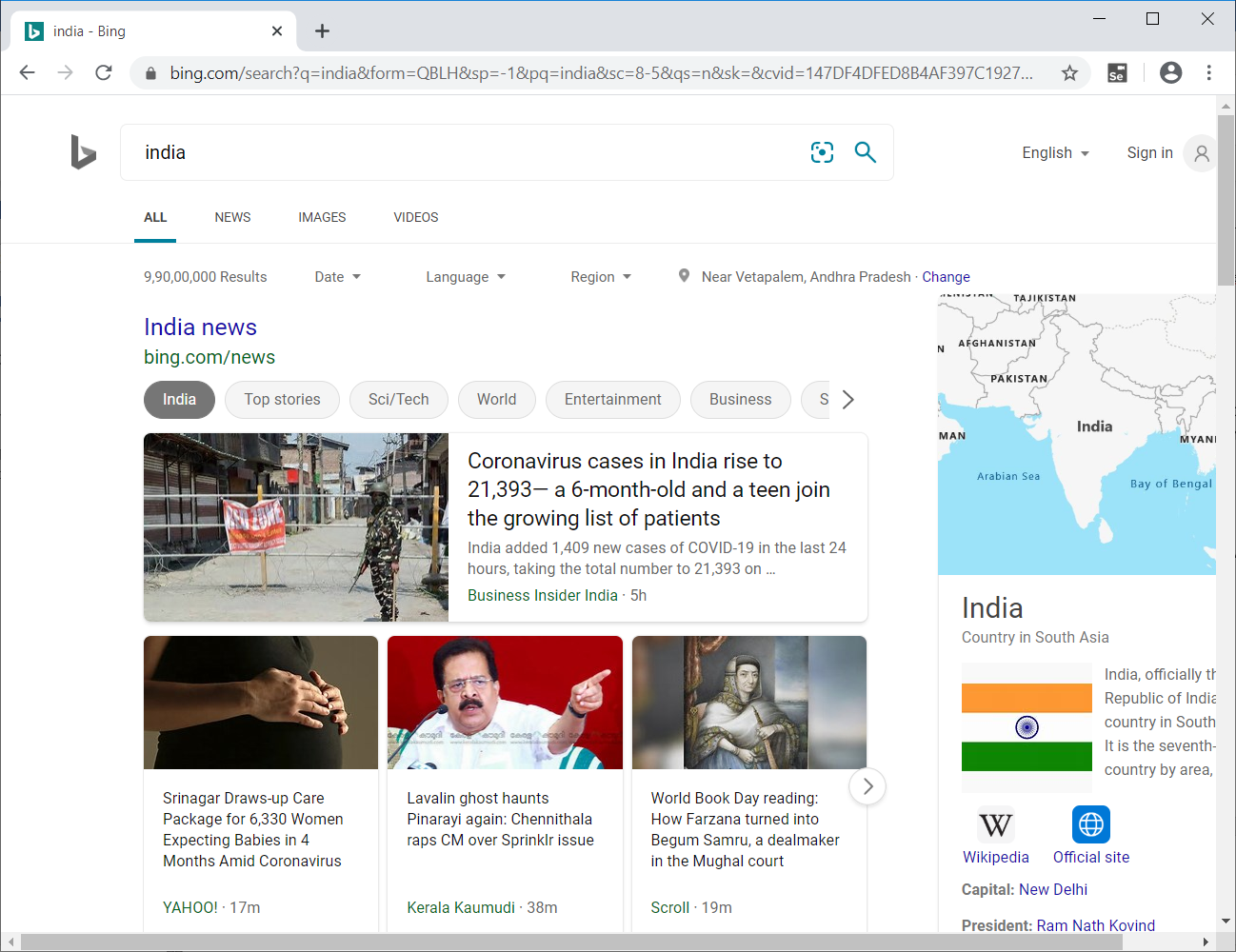
The above example gives a clear understanding of how relative xpath locator in Selenium works.
Relative Xpath Types
Relative XPath can be classified into below types -
- Standard Xpath
- Using Contains
- Using Xpath with AND & OR
- Using starts-with
- Using text in Xpath
Standard XPath
This method is used to find the element by the tag name and attribute value.
The syntax of the standard Xpath is
Xpath: //tagname[@attribute=’value’]
Here tagname indicates the tag, for example an input tag or anchor tag etc.
Example
Now, let’s understand the working of standard xpath locator with the help of a simple example. I will launch Chrome and navigate to bing.com. Here, I will try to locate the search box using standard xpath Locator.
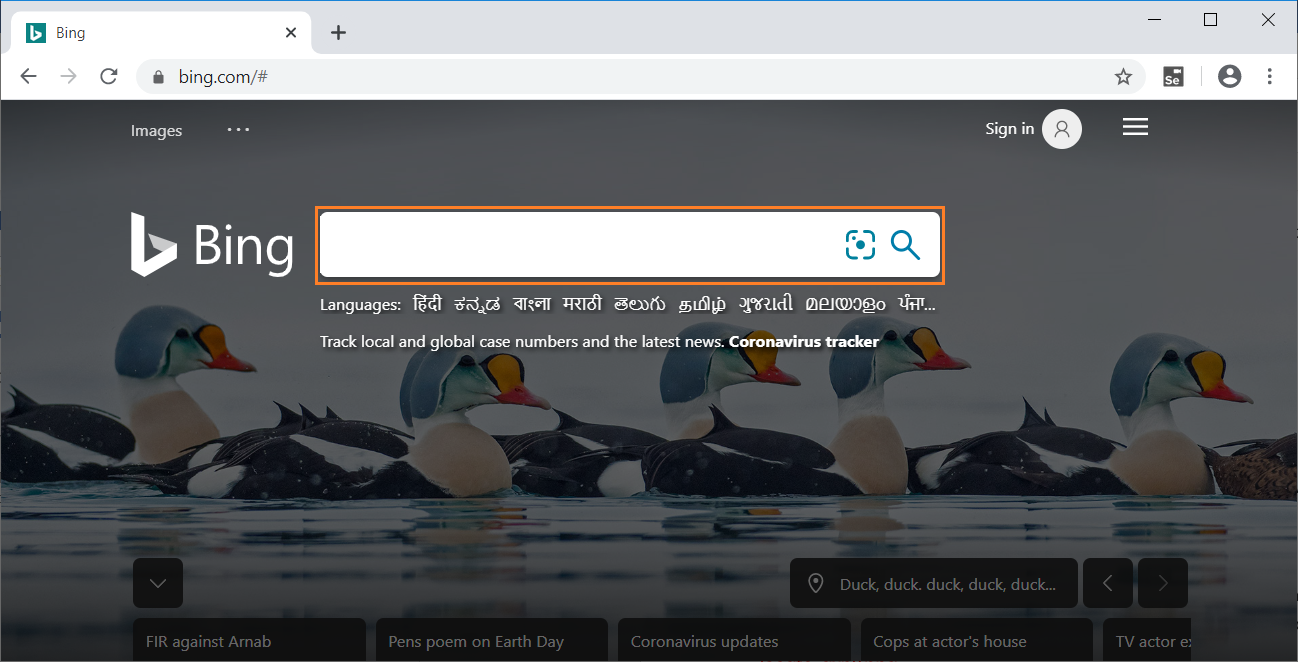
On inspecting the above web element, we can see an input tag has attribute id. Now, I will use the value of id i.e. "sb_form_q" along with standard xpath locator to perform search.
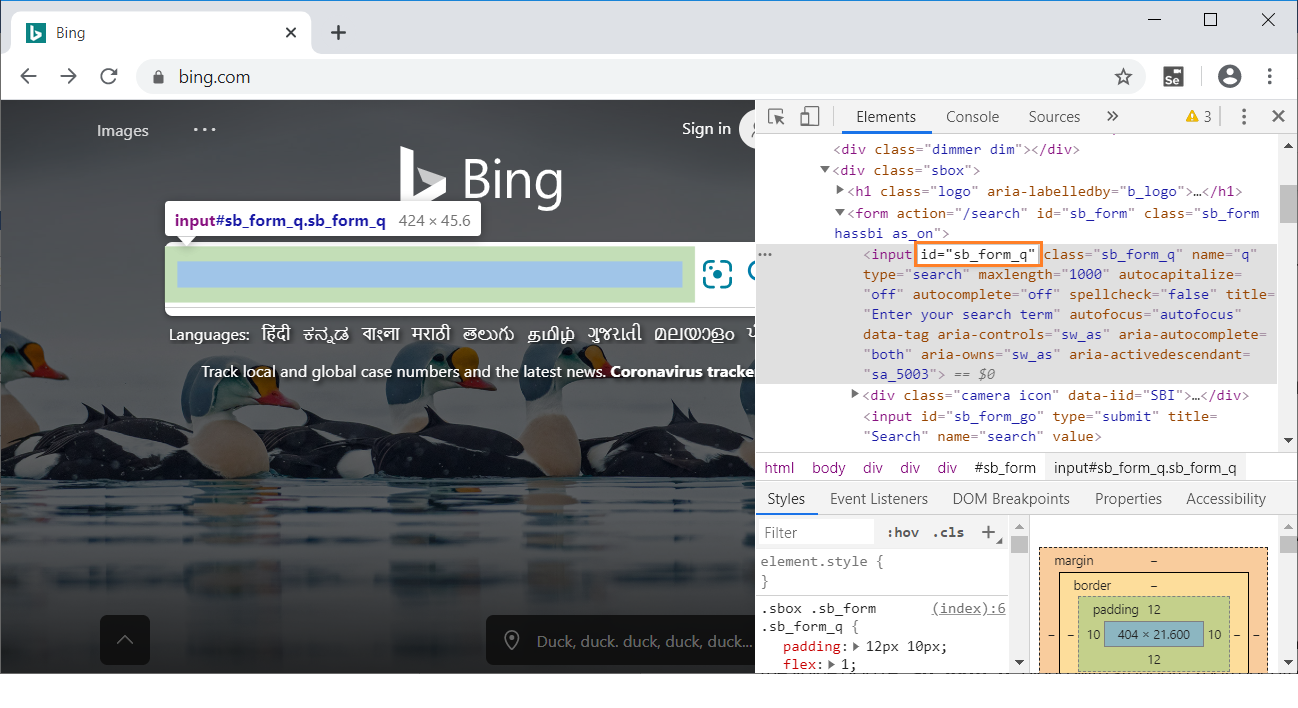
Let’s see how the automation of the search look like in Selenium IDE and send value "India" to the search box using id standard xpath locator.
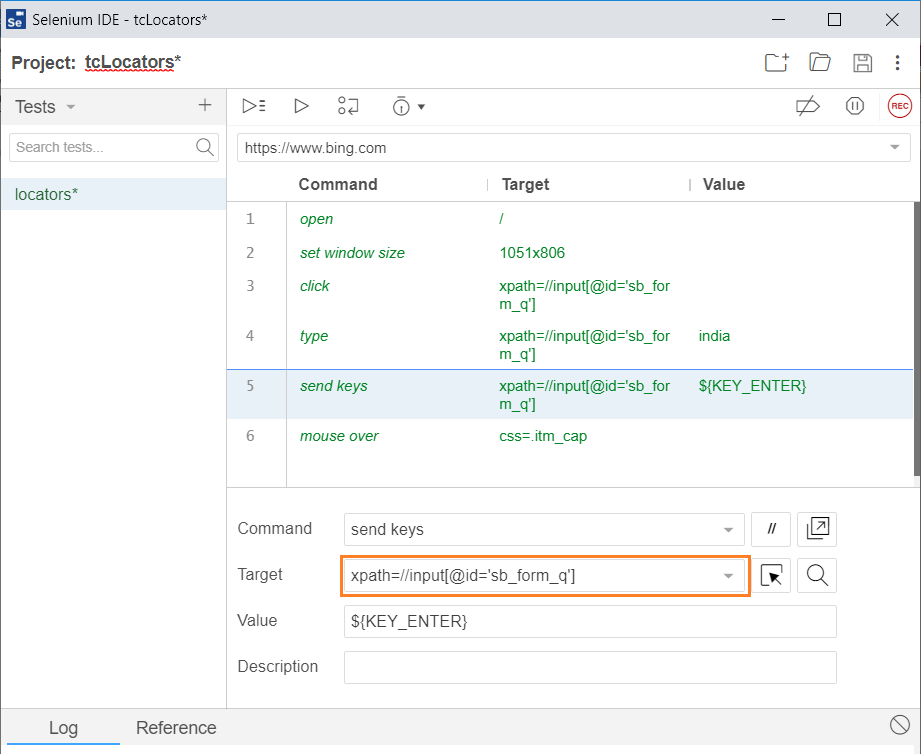
The java program for the above test is -
// Generated by Selenium IDE
import org.junit.Test;
import org.junit.Before;
import org.junit.After;
import static org.junit.Assert.*;
import static org.hamcrest.CoreMatchers.is;
import static org.hamcrest.core.IsNot.not;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.remote.RemoteWebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.Dimension;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.interactions.Actions;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.Alert;
import org.openqa.selenium.Keys;
import java.util.*;
import java.net.MalformedURLException;
import java.net.URL;
public class LocatorsTest {
private WebDriver driver;
private Map<String, Object> vars;
JavascriptExecutor js;
@Before
public void setUp() {
driver = new ChromeDriver();
js = (JavascriptExecutor) driver;
vars = new HashMap<String, Object>();
}
@After
public void tearDown() {
driver.quit();
}
@Test
public void locators() {
driver.get("https://www.bing.com/");
driver.manage().window().setSize(new Dimension(1051, 806));
driver.findElement(By.xpath(
"//input[@id=\'sb_form_q\']")).click();
driver.findElement(By.xpath(
"//input[@id=\'sb_form_q\']")).sendKeys("india");
driver.findElement(By.xpath(
"//input[@id=\'sb_form_q\']")).
sendKeys(Keys.ENTER);
{
WebElement element = driver.findElement(
By.cssSelector(".itm_cap"));
Actions builder = new Actions(driver);
builder.moveToElement(element).perform();
}
}
}
When we run the java program, Chrome driver launches chrome, redirect to bing search page, enter the search key word as "india" and navigate to search results page. Refer the below image for the output -
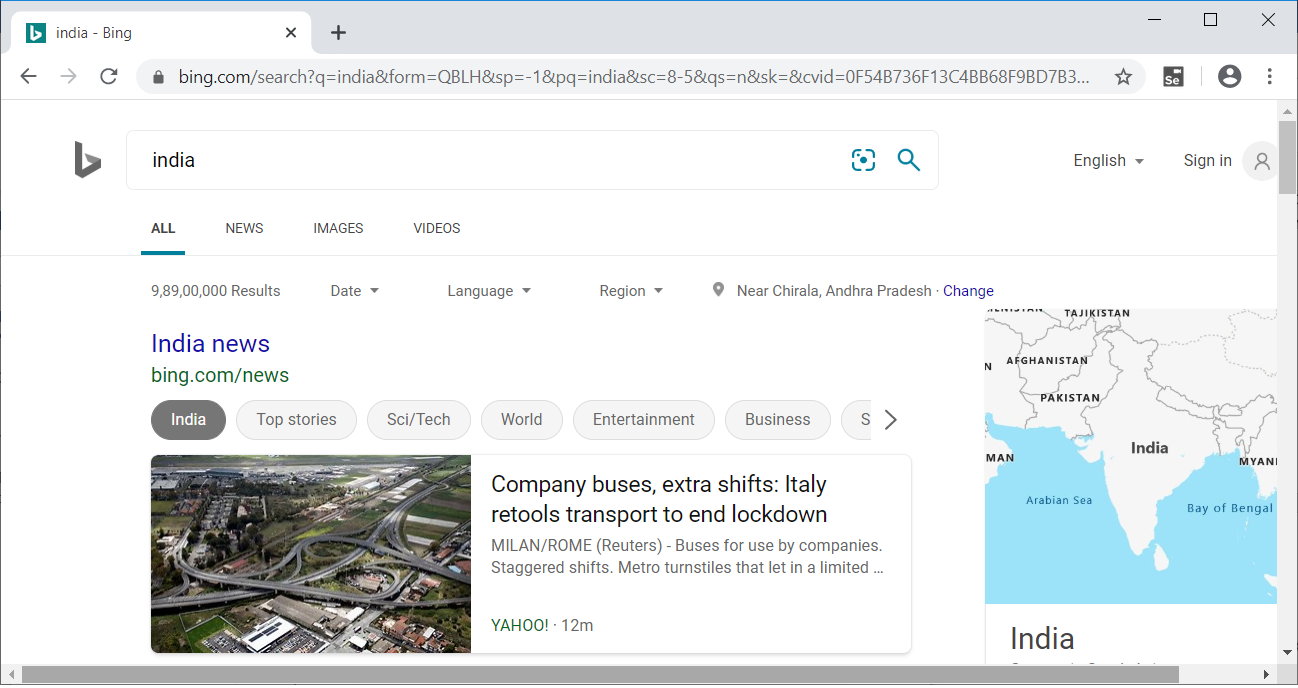
The above example gives a clear understanding of how standard xpath locator in Selenium works.
Contains
Contains works similar to CSS selector ‘contains’ symbol. Contains used when any element value changes dynamically and partially.
The syntax of the Xpath contains is -
Xpath: //tagname[contains(@attribute, ‘partial value of attribute’)]
Here tagname indicates the tag, for example an input tag or anchor tag etc.
Example
Now, let’s understand the working of contains xpath locator with the help of a simple example. I will launch Chrome and navigate to bing.com. Here, I will try to click on the gmail link using contains xpath Locator.
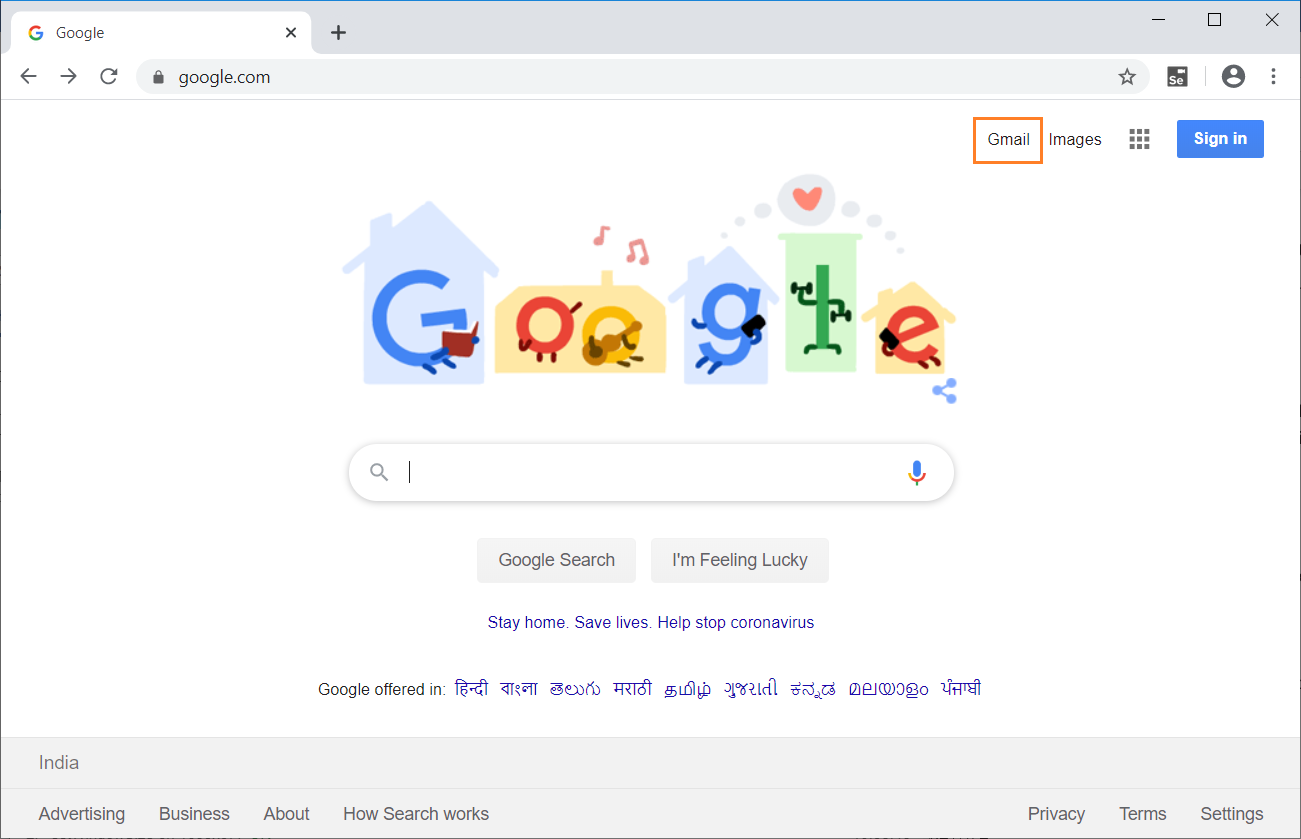
On inspecting the above web element, we can see an anchor tag has inner text "Gmail". Now, I will use the value of anchor tag i.e. "Gmail" with contains xpath locator to navigate to Gmail page.
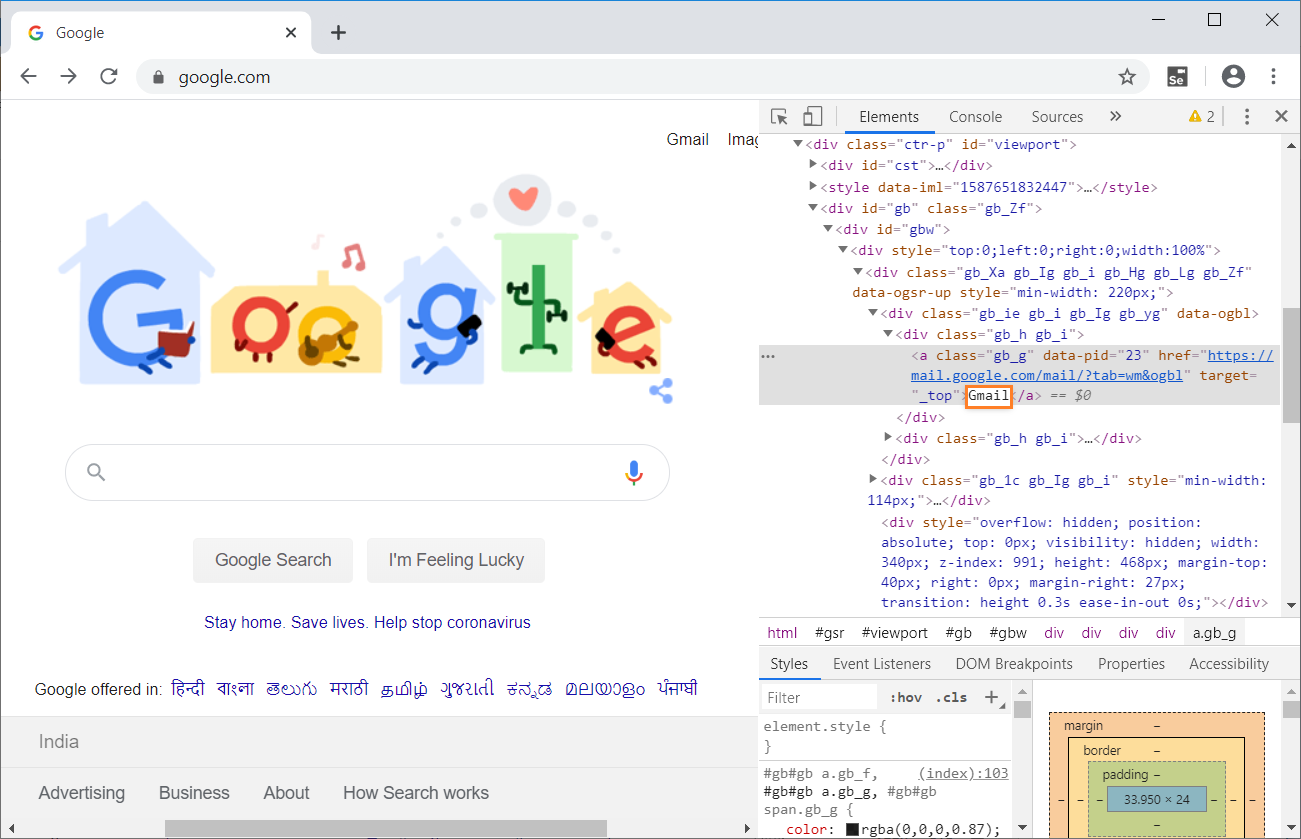
Let’s see how the automation of the gmail login navigation look like in Selenium IDE -
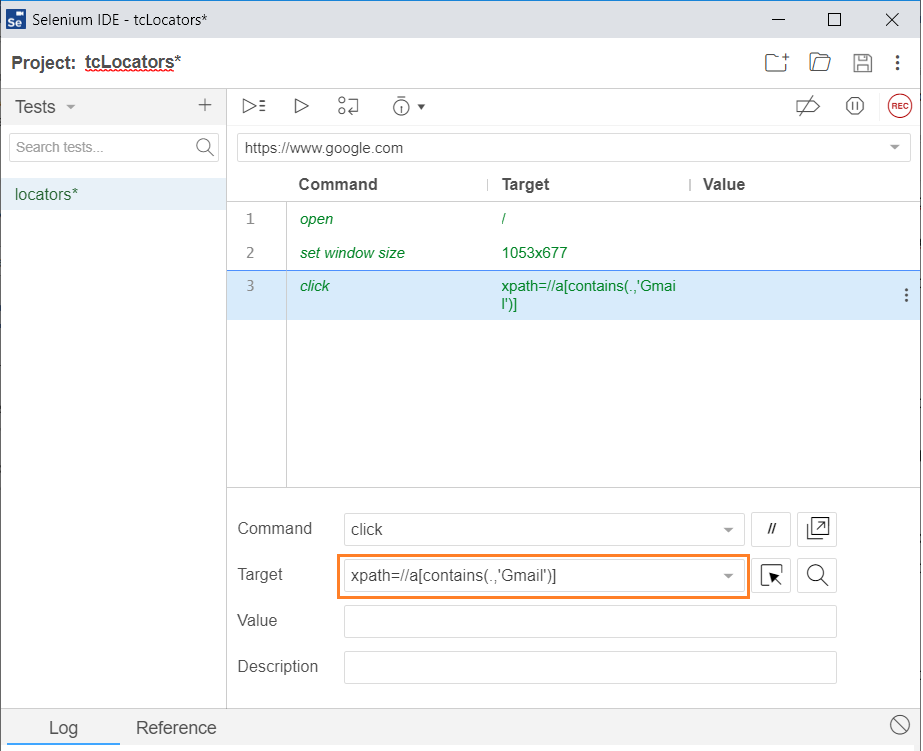
The java program for the above test is -
// Generated by Selenium IDE
// Generated by Selenium IDE
import org.junit.Test;
import org.junit.Before;
import org.junit.After;
import static org.junit.Assert.*;
import static org.hamcrest.CoreMatchers.is;
import static org.hamcrest.core.IsNot.not;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.remote.RemoteWebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.Dimension;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.interactions.Actions;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.Alert;
import org.openqa.selenium.Keys;
import java.util.*;
import java.net.MalformedURLException;
import java.net.URL;
public class LocatorsTest {
private WebDriver driver;
private Map<String, Object> vars;
JavascriptExecutor js;
@Before
public void setUp() {
driver = new ChromeDriver();
js = (JavascriptExecutor) driver;
vars = new HashMap<String, Object>();
}
@After
public void tearDown() {
driver.quit();
}
@Test
public void locators() {
driver.get("https://www.google.com/");
driver.manage().window().setSize(
new Dimension(1053, 677));
driver.findElement(By.xpath(
"//a[contains(.,\'Gmail\')]")).click();
}
}
When we run the java program, Chrome driver launches chrome, redirect to google search page, clicks on "Gmail" link and navigate to gmail navigation page. Refer the below image for the output -
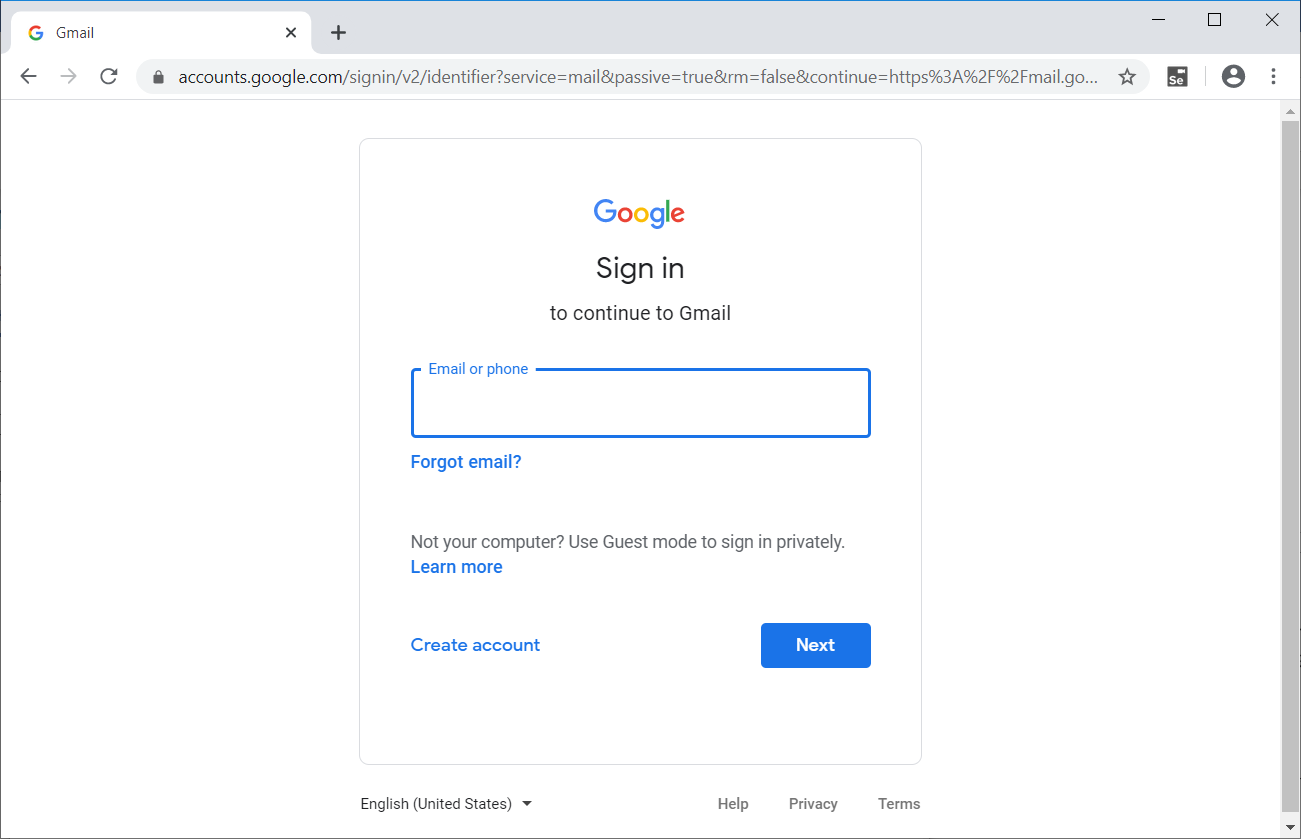
The above example gives a clear understanding of how contains xpath locator in Selenium works.
Xpath using ‘AND’ & ‘OR'
Xpath with logical operators used to incorporate multiple Xpath conditions at the same time. These are used to locate an element via two condition sets - ‘AND’ is for both the conditions should be true, ‘OR’ is for either of the two should be true.
Syntax using OR -
Xpath: //tagname[@attribute1=’value1’ OR @attribute2=’value2’]
Syntax using AND -
Xpath: //tagname[@attribute1=’value1’ AND @attribute2=’value2’]
Starts-with
starts-with is like the CSS selector. starts-with helps in locating element that starts with a specified attribute value.
The syntax of the Xpath starts-with is -
Xpath=//tagname[starts-with(@attribute=’starting name of the attribute value')]
Text
Text helps to locate element via xpath using exact text match. Most of the cases, we may have tags containing text that can be used to locate element.
The syntax of the Xpath text is -
Xpath=//tagname[text(),'Text used in the tagname']
Example
Now, let’s understand the working of text xpath locator with the help of a simple example. I will launch Chrome and navigate to google.com. Here, I will try to click on the gmail link using text xpath Locator.
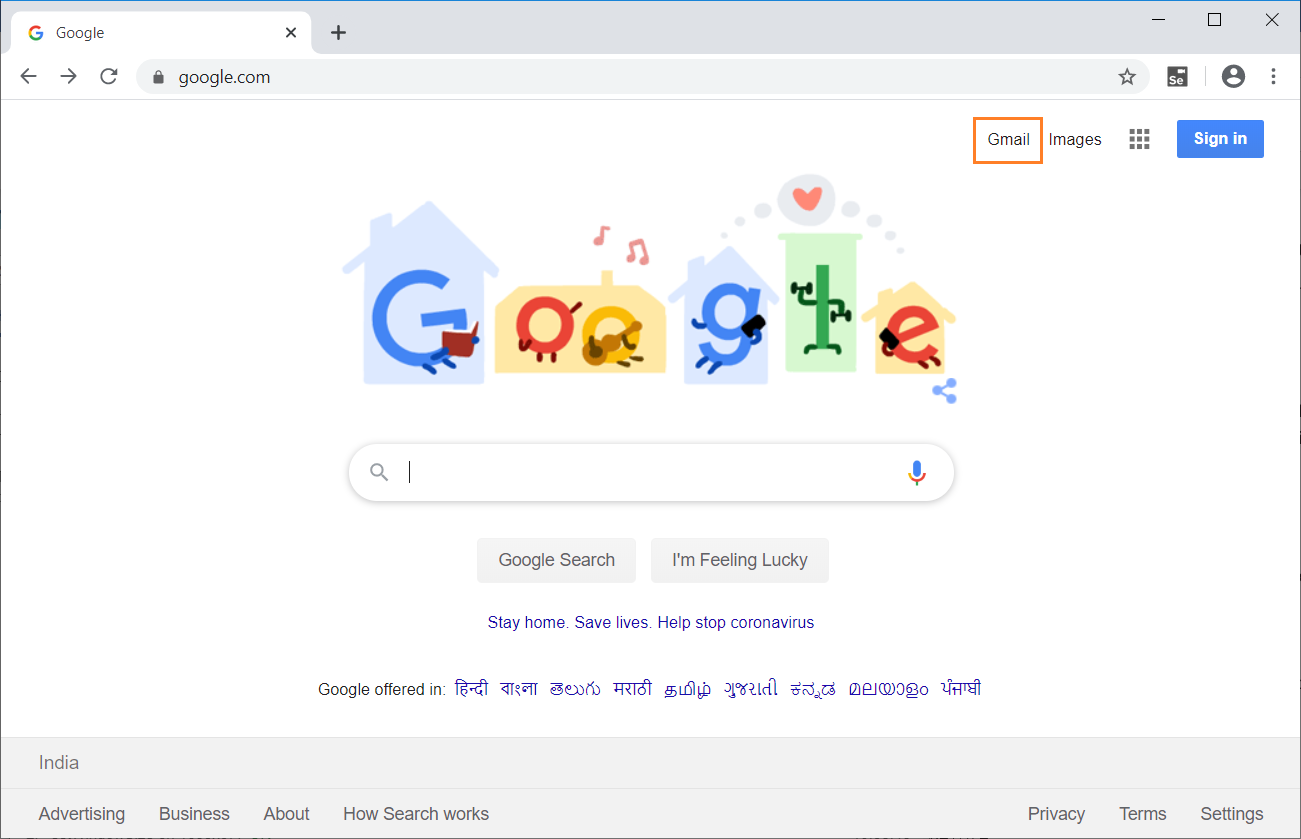
On inspecting the above web element, we can see an anchor tag has text "Gmail". Now, I will use the value of anchor tag i.e. "Gmail" with contains xpath locator to navigate to Gmail page.
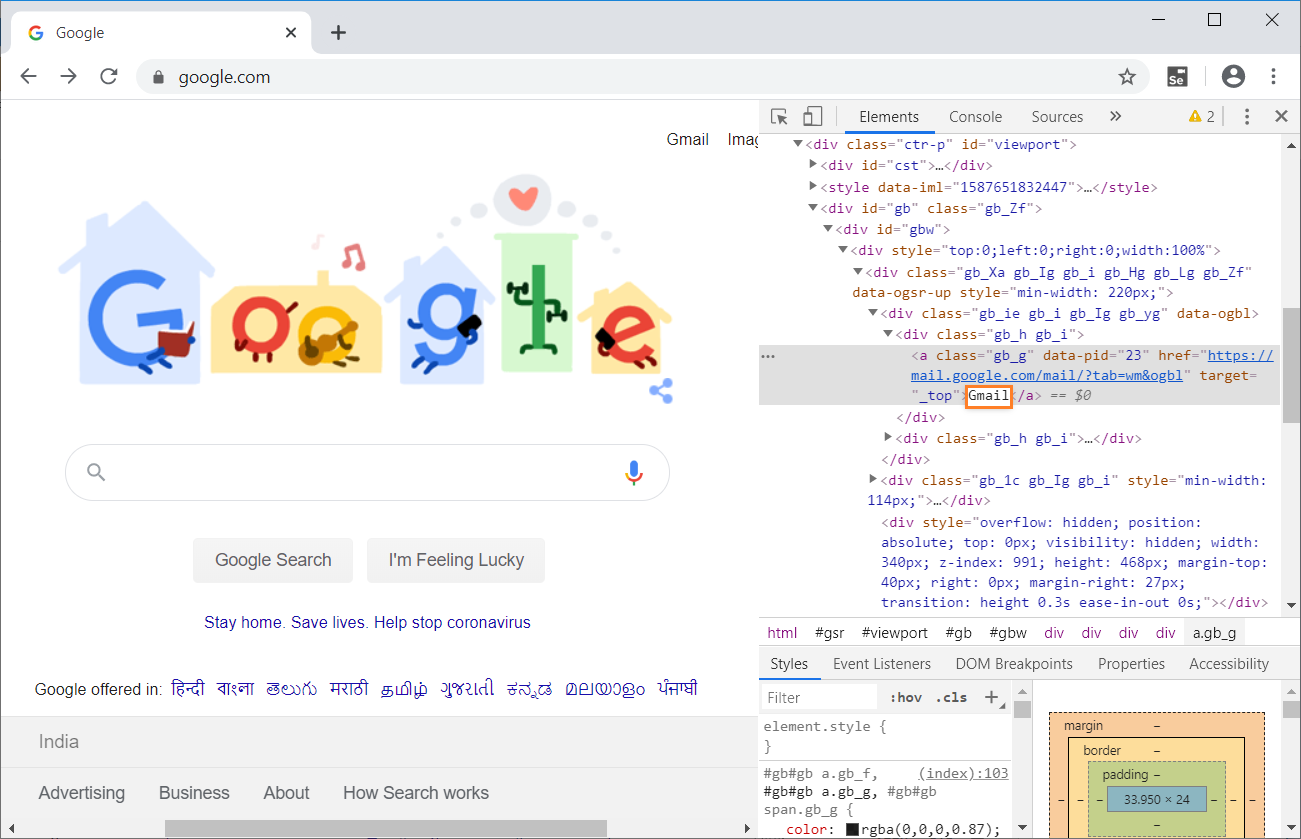
Let’s see how the automation of the gmail login navigation look like in Selenium IDE -
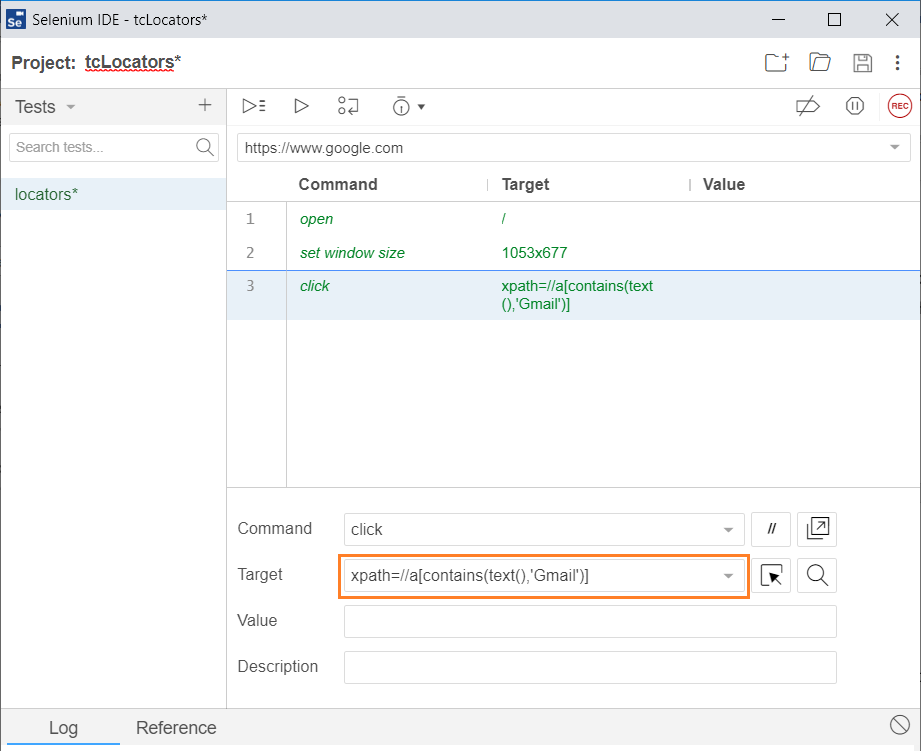
The java program for the above test is -
// Generated by Selenium IDE
// Generated by Selenium IDE
import org.junit.Test;
import org.junit.Before;
import org.junit.After;
import static org.junit.Assert.*;
import static org.hamcrest.CoreMatchers.is;
import static org.hamcrest.core.IsNot.not;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.remote.RemoteWebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.Dimension;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.interactions.Actions;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.Alert;
import org.openqa.selenium.Keys;
import java.util.*;
import java.net.MalformedURLException;
import java.net.URL;
public class LocatorsTest {
private WebDriver driver;
private Map<String, Object> vars;
JavascriptExecutor js;
@Before
public void setUp() {
driver = new ChromeDriver();
js = (JavascriptExecutor) driver;
vars = new HashMap<String, Object>();
}
@After
public void tearDown() {
driver.quit();
}
@Test
public void locators() {
driver.get("https://www.google.com/");
driver.manage().window().setSize(new Dimension(1053, 677));
driver.findElement(By.xpath(
"//a[contains(text(),\'Gmail\')]")).click();
}
}
When we run the java program, Chrome driver launches chrome, redirect to google search page, clicks on "Gmail" link and navigate to gmail navigation page. Refer the below image for the output
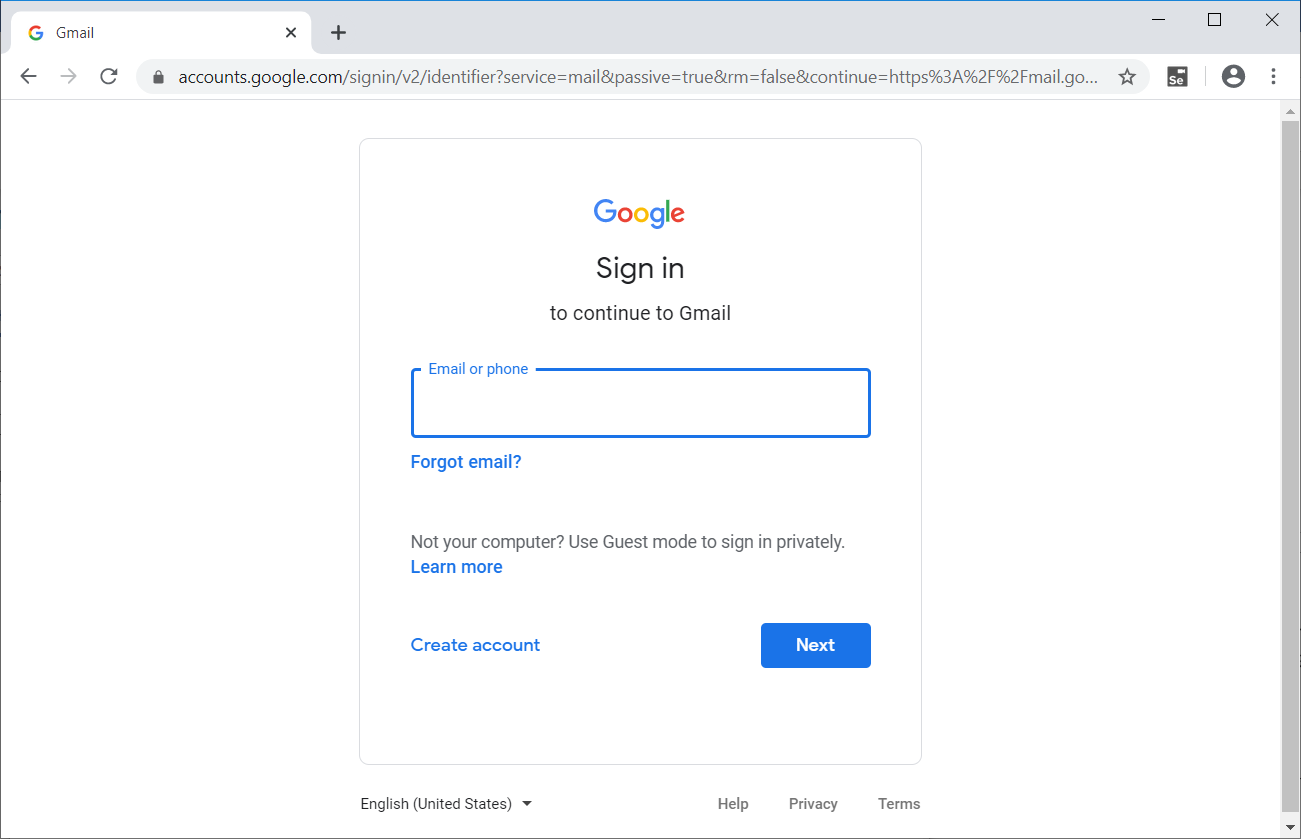
The above example gives a clear understanding of how text xpath locator in Selenium works .