Summary -
In this topic, we described about the below sections -
What is Exception?
Exception is an runtime errors that occurs during the program execution. The runtime errors can be of two types -
- Catchable Runtime Errors which can be caught using the exception handling.
- Non-Catchable Runtime Errors which always results the termination of program.
Normally exceptions might occur due to performing an invalid operation or invalid movements. Exception disturbs the normal flow of the program execution.
Due to the exception, the program execution terminates abnormally which is not expected. The exceptions need to be handled to skip the termination of the program and get the expected result.
Exception Categories -
Exceptions are represented by objects that are instances of classes. All those type of classes are called as exception classes. cx_root is the root class of all the exception classes and the major classes inherited from this class.
The root class is used to define handlers for all types of exceptions. The super class cx_root derives three major classes and those are -

- CX_STATIC_CHECK – Used for the exception handler static protection. Exceptions of this category must be declared explicitly in the procedure interface. It is verified by both the compiler and runtime system.
- CX_DYNAMIC_CHECK – Used for error situations that can be prevented by preconditions. Exceptions of this category should also declare explicitly in the procedure interface. It is verified by runtime system only.
- CX_NO_CHECK – Used for situations that cannot handled immediately. Exceptions of this category cannot be declared explicitly in the procedure interface.
Exception Attributes & Methods -
Exception clauses inherit the below attributes and methods from cx_root -
Attributes & Methods | Description |
---|---|
Textid | This attribute used to define different texts for exceptions. Effects the result of the method get_text. |
Previous | This attribute can store the original exception which allows us to build a chain of exceptions. |
get_text | This method returns the textual representation as a string according to the exception system language. |
get_longtext | This method returns the long variant of the exception textual representation as a string. |
get_source_position | This method returns the program name, include name and line number where the exception was raised. |
What is Exception Handling?
ABAP supports exception handling for handling the exceptions to get the expected results. Exception handling provides a way to transfer the control from error occuring code to another to avoid the program termination.
Exception handling can be achieved by using keywords TRY, CATCH and CLEANUP. Exceptions can be occured at any point of time by a misplaced/wrong piece of code in the program.
To handle the exception, the problemetic code that causes the error should code under TRY block. All the exception handling code should be coded in between TRY and ENDTRY block.
Exception are basically two types and those are -
- Exceptions raised during the program execution due to invalid calculations or invalid movements.
- Exceptions created by the programmer based on the requirements.
Syntax -
TRY.
*Write code block here which causes an exception
Code-that-causes-exception
CATCH [BEFORE UNWIND] EX_CLASS1.
Catch-block-for-class EX_CLASS1.
CATCH [BEFORE UNWIND] EX_CLASS2.
Catch-block-for-class EX_CLASS2.
.
.
.
CATCH [BEFORE UNWIND] EX_CLASSn.
Catch-block-for-class EX_CLASSn.
CATCH cx_root.
Catch-block-for-of-all-kinds-of-exceptions.
CLEANUP [INTO oref].
Cleanup-block-to-restore-the-consistent-state.
ENDTRY.
- TRY − The TRY block contains the problematic code that is expected to cause an exception. It may contain control structures and calls of procedures or other ABAP code.
- CATCH – The CATCH block executes when the specified exception in CATCH statement matched to the exception occurred. The CATCH block contains the statements to handle the exception.
- CLEANUP − The statements in the CLEANUP block are executed whenever an exception not caught by the handler of the same TRY ... ENDTRY construct. Within the CLEANUP clause, the system can restore an object to a consistent state or release external resources.
How many parts in Exception Handling?
Exceptions handling have two parts and those are -
- Raising the exception – This part used to identify the code that causes the exception and add the code to the TRY block.
- Catching the exception – Handlers used to catch the exception. In this part, identify the type of the exception and we have to code with CATCH block to handle the exception.
- Handlers should be defined for statements in a TRY block (TRY... ENDTRY).
- Within the TRY ENDTRY, each handler is stated by a CATCH statement followed by the exception class.
- The statements that causes the exception should code in between TRY and the first CATCH.
- The statements following the CATCH clause are executed only if the handler catches an exception.
- The CATCH block has no scope terminator and next CATCH statement or CLEANUP statement or ENDTRY act as scope terminators.
- Each exception is caught only once by the first suitable handler.
- Each handler can catch more than one type of exceptions based on the definition.
- The sequence of handlers is more important.
- If system cannot find an appropriate handler in a TRY ... ENDTRY construct, it searches for handlers in the next outer TRY ... ENDTRY construct. If it cannot find there, it continues the search upward in the call hierarchy.
- Each TRY ... ENDTRY construct contains a maximum of one CLEANUP clause.
Example -
Below example explains how the exception can be handled in ABAP programming. Program for multiplication two number with non-numeric exception handling.
Code -
*&---------------------------------------------------------------------*
*& Report Z_EXCEPTION_HANDLING
*&---------------------------------------------------------------------*
*& Written by TutorialsCampus
*&---------------------------------------------------------------------*
REPORT Z_EXCEPTION_HANDLING.
* Declaring variables
DATA: rslt TYPE p LENGTH 10,
op_ref TYPE REF TO cx_root,
msg_text TYPE string.
* Declaring parameters
PARAMETERS: inp1 TYPE c LENGTH 5,
inp2 TYPE c LENGTH 5.
* Try block starting
TRY.
* Result calculation by multiplying both inputs inp1, inp2.
rslt = inp1 * inp2.
* CATCH for cx_sy_conversion_no_number: Value for Attribute TEXTID
CATCH cx_sy_conversion_no_number INTO op_ref.
* moving the error mssage msg_text from op_ref->get_text( )
msg_text = op_ref->get_text( ).
* Default CATCH cx_root for any other exceptions.
CATCH cx_root INTO op_ref.
* moving the error mssage msg_text from op_ref->get_text( ).
msg_text = op_ref->get_text( ).
ENDTRY.
* Validating if any error occured.
IF NOT msg_text IS INITIAL.
* Displays only when error occured.
WRITE / msg_text.
ELSE.
* Displays only when no error occured.
WRITE: / 'Multiplication Result :', rslt.
ENDIF.
Output -
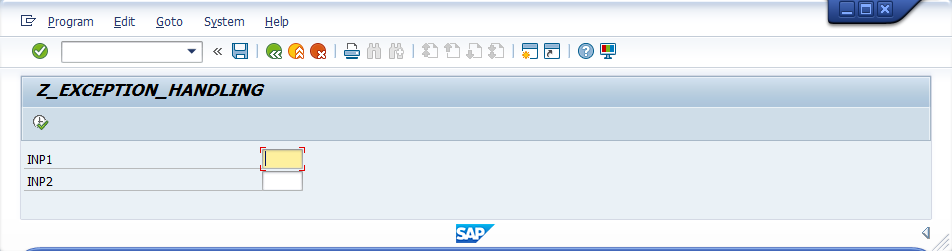
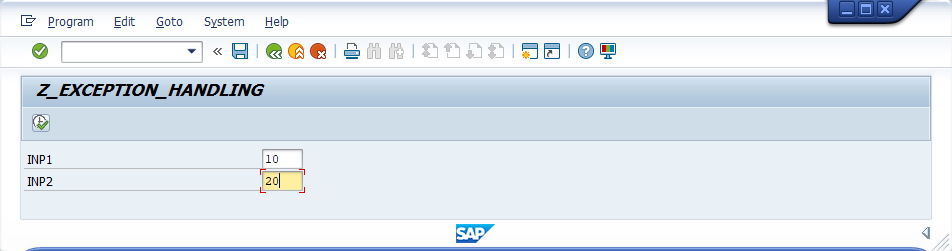
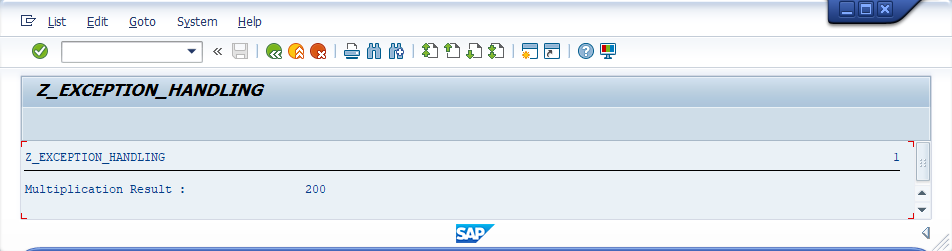
Case-2: Both inputs are not valid and non-numeric
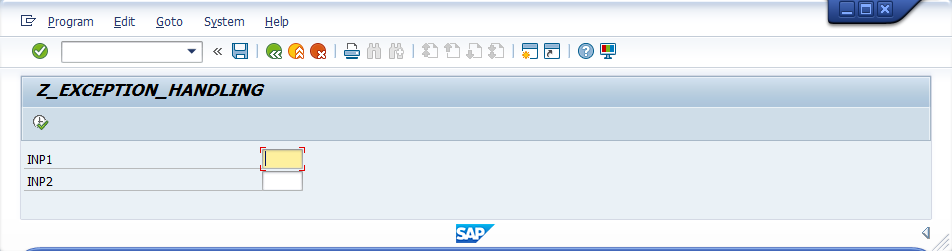
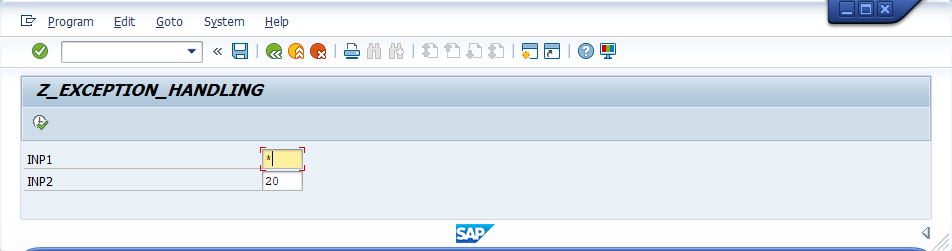

Explaining Example -
In the above example, each and every statement is preceeded with a comment to explain about the statement. Go through them to get clear understanding of example code.
TRY...ENDTRY represents the try block. rslt = inp1 * inp2 is the code that expects to causes the exception. CATCH cx_sy_conversion_no_number INTO op_ref, used to catch the cx_sy_conversion_no_number exception into op_ref.
msg_text = op_ref->get_text( ), moving the error mssage msg_text from op_ref->get_text( ). CATCH cx_root INTO op_ref, used to catch any other exceptions. IF NOT msg_text IS INITIAL, verifies the msg_text is initial or not. If it is initial, displays the error from msg_text. Otherwise, multiplication result rslt.