Summary -
In this topic, we described about the below sections -
In addition to these built-in types, the ABAP supports two Dictionary types TIMESTAMP and TIMESTAMPL. These are used in many standard application tables and to store a timestamp in the UTC format.
"UTC" ("Universal Time Consolidated") is a time standard based on the International Atomic Time standard. These standards define a global time standard that can be used to convert a given time to local time and vice versa.
Data type Keyword | Length | Description |
---|---|---|
TIMESTAMP | Type P – Length 8 No decimals | Used to represent short timestamps. Format is YYYYMMDDhhmmss. |
TIMESTAMPL | Type P - Length 11 Decimals 7 | used to represent long timestamps. Format is YYYYMMDDhhmmssmmmuuun. mmmuuun represent fractions of a second. |
Working with Timestamp -
TIMESTAMP and TIMESTAMPL are two Dictionary types to work with timestamps. These two types are used to store time sensitive data.
ABAP provides some support to these types in the form of some built-in functions/statements. CL_ABAP_TSTMP is one of the built-in function used to simplify the process of working with timestamps.
GET TIME STAMP is used to get the current system time. GET TIME STAMP can store the timestamp in the shorthand or longhand format based on the target variable declaration.
SY-ZONLO used to display the timestamp in the local time zone configured in the user preferences.
Example -
Below example shows timestamp usage in ABAP application program.
Code -
*&---------------------------------------------------------------------*
*& Report Z_TIMESTAMPS
*&---------------------------------------------------------------------*
*& Written by TutorialsCampus
*&---------------------------------------------------------------------*
REPORT Z_TIMESTAMPS.
* Declaring variables of type timestamp.
DATA: W_STS TYPE TIMESTAMP,
W_LTS TYPE TIMESTAMPL.
*Assigning timestamp to short timestamp variable
GET TIME STAMP FIELD W_STS.
WRITE: / 'Shorthand TimeStamp : ', W_STS TIME ZONE SY-ZONLO.
*Assigning timestamp to long timestamp variable
GET TIME STAMP FIELD W_LTS.
WRITE: / 'Longhand TimeStamp : ', W_LTS TIME ZONE SY-ZONLO.
Output -

Explaining Example -
In the above example, each and every statement is preceeded with a comment to explain about the statement. Go through them to get clear understanding of example code.
GET TIME STAMP FIELD W_STS, sets the short timestamp value to W_STS field. GET TIME STAMP FIELD W_LTS, sets the long timestamp value to W_LTS field.
W_LTS TIME ZONE SY-ZONLO, displays the timestamp using TIME ZONE SY-ZONLO.
Converting Date & Time to Timestamp or vice versa -
Assume date and time is only available, but the target value required was timestamp. In this case, the date and time needs to be converted/combined as a timestamp.
In other way, Assume if only timestamp is available but the date and time required separately. In this case, the timestamp should be split into date and time.
ABAP supports converting the below scenarios. Those are -
- Timestamp from date & time
- Split timestamp to date & time
Syntax - Timestamp from date & time:
CONVERT DATE w_date [TIME w_time [DAYLIGHT SAVING TIME w_dst]]
INTO TIME STAMP w_tstamp TIME ZONE w_tzone.
Syntax – Split timestamp to date & time:
CONVERT TIME STAMP w_tstamp TIME ZONE w_tzone INTO
[ DATE w_date] [ TIME w_time ] [DAYLIGHT SAVING TIME w_dst].
Example -
Below example shows timestamp conversion in ABAP application program.
Code -
*&---------------------------------------------------------------------*
*& Report Z_CONV_TIMESTAMPS
*&---------------------------------------------------------------------*
*& Written by TutorialsCampus
*&---------------------------------------------------------------------*
REPORT Z_CONV_TIMESTAMPS.
* Declaring required variables
DATA: W_DATE1 TYPE D,
W_DATE2 TYPE D,
W_TIME1 TYPE T,
W_TIME2 TYPE T,
W_STS TYPE TIMESTAMP,
W_LTS TYPE TIMESTAMPL.
* Assigning current date to W_DATE1
W_DATE1 = SY-DATUM.
WRITE: / 'Current Date W_DATE1 : ', W_DATE1 DD/MM/YYYY.
* Assigning current time to W_TIME1
W_TIME1 = SY-UZEIT.
WRITE /(60) W_TIME1 USING EDIT MASK 'The current time is __:__:__'.
* Converting date & time to create timestamp
CONVERT DATE W_DATE1 TIME W_TIME1
INTO TIME STAMP W_LTS TIME ZONE SY-ZONLO.
WRITE: / 'Converted TimeStamp from Date and time W_LTS: ',
W_LTS TIME ZONE SY-ZONLO.
* Assigning current timestamp to W_STS
GET TIME STAMP FIELD W_STS.
WRITE: / 'Current TimeStamp W_STS : ', W_STS TIME ZONE SY-ZONLO.
* Splitting timestamp to date & time
CONVERT TIME STAMP W_STS TIME ZONE SY-ZONLO
INTO DATE W_DATE2 TIME W_TIME2.
WRITE: / 'Converted Date W_DATE2: ', W_DATE2 DD/MM/YYYY.
WRITE /(60) W_TIME2 USING EDIT MASK 'The converted time is __:__:__'.
Output -
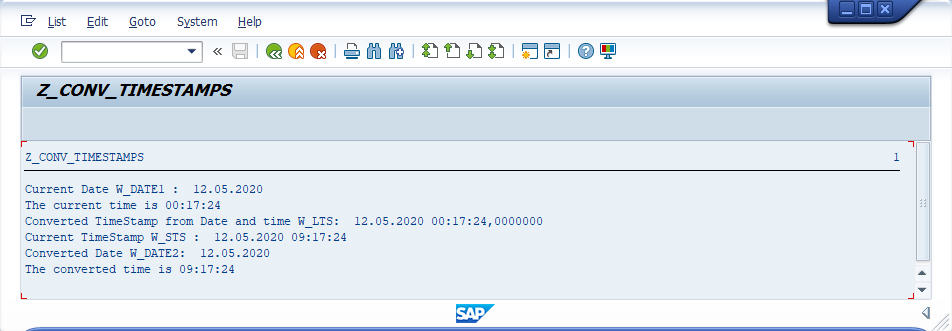
Explaining Example -
In the above example, each and every statement is preceeded with a comment to explain about the statement. Go through them to get clear understanding of example code.
CONVERT DATE W_DATE1 TIME W_TIME1 INTO TIME STAMP W_LTS TIME ZONE SY-ZONLO, converts date W_DATE1 and time W_TIME1 into timestamp W_LTS of timezone SY-ZONLO.
CONVERT TIME STAMP W_STS TIME ZONE SY-ZONLO INTO DATE W_DATE2 TIME W_TIME2, converts time stamp W_STS of time zone SY-ZONLO into date W_DATE2 and time W_TIME2.