Summary -
In this topic, we described about the below sections -
ABAP program is set of processing blocks. Each processing block contains one or more statements. The Syntax of ABAP programming language consists of the following –
- Statements
- Formatting ABAP statements (Indentation)
- Chained statement or colon notation
- Comments
ABAP Statements –
ABAP program contains individual ABAP statements. Each statement begins with a keyword and ends with a period(.). ABAP statements explained in detail here .
Formatting ABAP statements (Indentation) –
ABAP statement coding has no restriction. We can enter ABAP statement in any format. i.e. Multiple ABAP statements can be written on single line. Single ABAP statement can be split into multiple lines.
Original Program fragment -
PROGRAM Z_FIRST_PROG.
WRITE 'Hello World, Welcome to TutorialsCampus'.
Could also written as –
PROGRAM Z_FIRST_PROG. WRITE 'Hello World, Welcome to TutorialsCampus'.
Or as follows –
PROGRAM
Z_FIRST_PROG.
WRITE
'Hello World, Welcome to TutorialsCampus'.
The elements of a statement should be separated by at least one blank/space or a line break. Otherwise, blanks and line breaks between tokens are not significant.
Below examples shows the valid and invalid statements -

Colon notation or Chain statement –
ABAP programming allows to concatenate consecutive statements with same keyword in the first part into a chain statement.
If any two or more statements started with same keywords coded consecutively together or one after the other, ABAP has a feasibility to combine them in a single statement. To combine two or more consecutive statements, colon (:) should be used after the keyword.
To concatenate sequence of statements, write the identical keyword only once and place a colon(:) immediately after keyword. After colon(:), write the remaining parts of the statements one after the other with a comma (,) separated. After all written, place a period (.) at the end of the statement.
Syntax -
ABAP_keyword : statements-comma-seperated.
For example, the declaration statement sequence shown below -
DATA v_a TYPE I.
DATA v_b TYPE P.
Chained statement -
DATA: v_a TYPE I, v_b TYPE P.
In the chain statement, after the colon the remaining parts of the statements are comma separated. We can write the statement like below -
DATA: v_a TYPE I,
v_b TYPE P.
In a chain statement, the first part (before the colon) is not limited to the keyword of the statements. The identical part of all the statements can code before the colon(:).
For example, the processing statement sequence shown below –
SUM = SUM + 1.
SUM = SUM + 2.
SUM = SUM + 3.
Chain statement for the above –
SUM = SUM + : 1, 2, 3.
In the above example, "SUM = SUM + " is the identical part of all the ABAP statements. So to make the chain statement for the above, write the common part first, next code colon(:) and then remaining part of statements comma separated.
Example -
Problem should be described here
Code -
*&---------------------------------------------------------------------*
*& Report Z_COLON_NOTATION
*&---------------------------------------------------------------------*
*& Written by TutorialsCampus
*&---------------------------------------------------------------------*
REPORT Z_COLON_NOTATION.
* Displaying 'Hello ' on the output.
WRITE 'Hello '.
* Displaying 'World..!' on the same row of the output.
WRITE 'World..!'.
* Skipping next two lines on the output.
SKIP 2.
* Chained statement
WRITE : 'Hello ', 'World..!'.
Output -

Explaining Example -
In the above example, each and every statement is preceeded with a comment to explain about the statement. Go through them to get clear understanding of example code.
WRITE 'Hello '.
WRITE 'World..!'. - write output on the line 3 and 4. SKIP 2 - Inserts 2 empty line and places the cursor on 6th line.
WRITE : 'Hello ', 'World..!' - Writes the output on the line 6. First two WRITE statements equal to third WRITE statement.
Comments –
Comments or Non-executable statements definition are self-explanatory. Comments generally used to understand the code easily for the programmers. Comments are do nothing statements and are ignored by the computer.
There are two types of comments that are supported in ABAP editor and those are -
- Full line comments or Comment Lines
- Partial comments or End of line comments
Full line comments or Comment Lines -
Full line comment contains only a comment for the full line and nothing else.
Full line comments can be made by using asterisk (*) or quote(") as a first character of the entire line. If the line starts with * or ", then those lines are considered as comments and ignored by the compiler while generating program.
For example, let us write a full line comment below –
* Full line comment in SAP.
Partial comments or End of line comments –
If user creates a comment at the end of the executable statement (after a period), those called as a partial comment. Partial comment should get started with double quote (“). If any information starts with “, then those are ignored by the compiler while generating program. Otherwise, compilation errors occurred.
For example, let us write a partial comment below –
Write 'Hello World..!'. "Starting of a Partial comment
Example -
Write a program to show case full and partial comments.
Code -
*&---------------------------------------------------------------------*
*& Report Z_COMMENTS
*&---------------------------------------------------------------------*
*& Written by TutorialsCampus
*&---------------------------------------------------------------------*
REPORT Z_COMMENTS.
* Full line comment
WRITE 'Hello World..!'. "partial comment
Output -
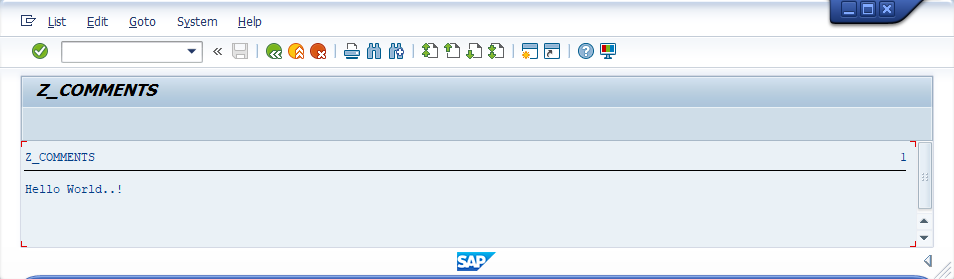
Explaining Example -
In the above example, each and every statement is preceeded with a comment to explain about the statement. Go through them to get clear understanding of example code.
* Full line comment - Full line comment in the program. " partial comment - Partial line comment in the program.